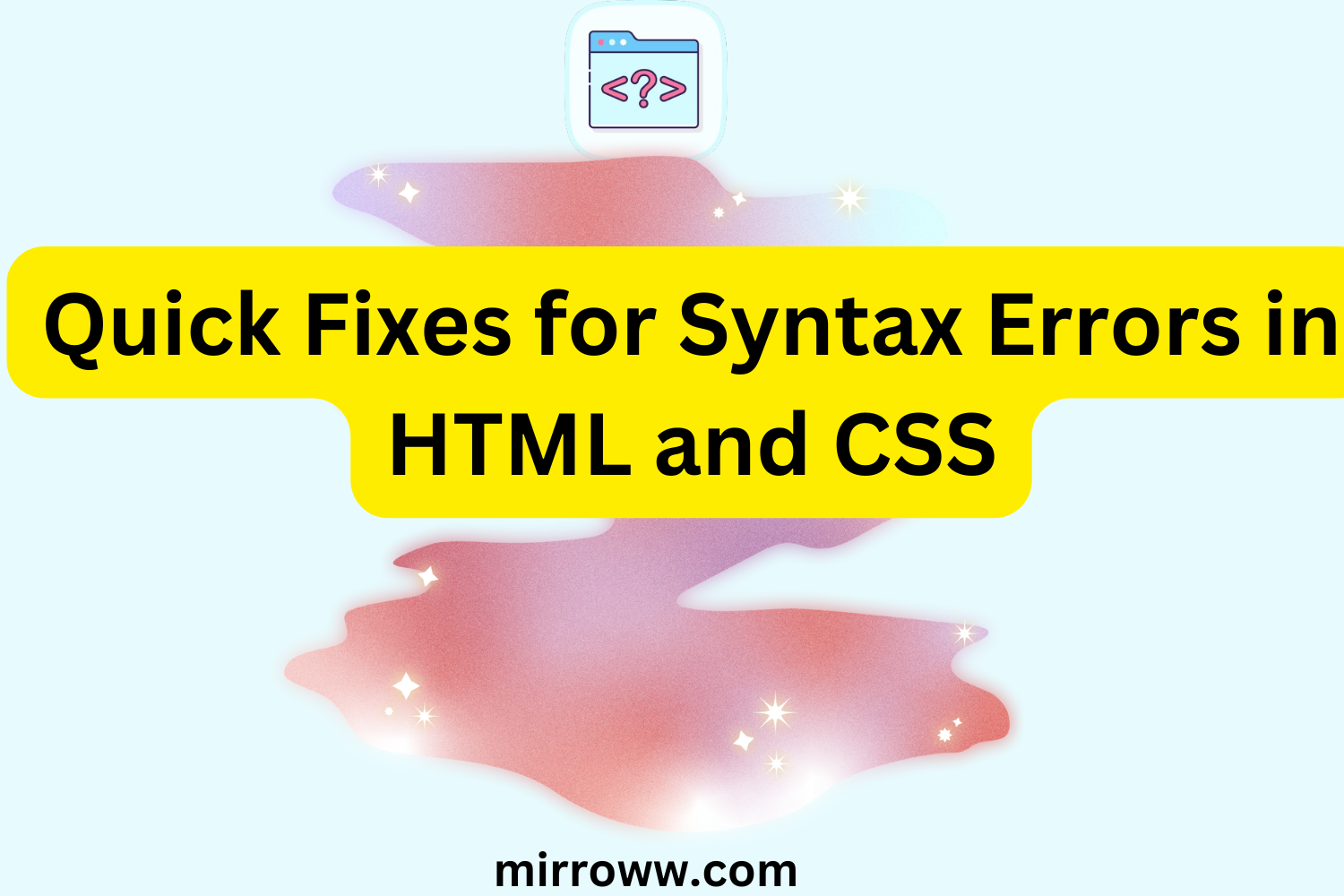
Quick Fixes for Syntax Errors in HTML and CSS
Web development is both an art and a science, and syntax errors are its persistent villains. They sneak into your code, wreaking havoc on layouts and functionality. Don’t worry; this article dives into quick fixes for common HTML and CSS syntax errors—all while keeping things engaging, practical, and optimized for search engines.
Why Syntax Errors Matter
Syntax errors are like typos in your recipe. A missing ingredient or misstep could turn a culinary masterpiece into a disaster. Similarly, even minor HTML and CSS errors can:
- Break website layouts
- Affect user experience (UX)
- Harm search engine optimization (SEO) rankings
- Make debugging a nightmare
In short, syntax errors disrupt your website’s ability to function smoothly and rank well. Let’s tackle these issues head-on.
Common HTML Syntax Errors and Fixes
1. Missing or Mismatched Tags
HTML relies on properly nested tags. A single missing or mismatched tag can break the entire document structure.
Example Error:
<div>
<p>This is a paragraph.</div>
Fix: Ensure every opening tag has a corresponding closing tag.
<div>
<p>This is a paragraph.</p>
</div>
Pro Tip: Use a code editor with syntax highlighting or a validation tool like W3C Validator.
2. Unquoted Attribute Values
HTML attributes must be enclosed in quotes.
Example Error:
<img src=path/to/image.jpg alt=Example image>
Fix: Add double or single quotes around attribute values.
<img src="path/to/image.jpg" alt="Example image">
3. Improperly Nested Elements
HTML must follow strict nesting rules.
Example Error:
<b><i>Bold and italic</b></i>
Fix: Close the inner tag before the outer tag.
<b><i>Bold and italic</i></b>
4. Forgotten DOCTYPE Declaration
Without the DOCTYPE declaration, browsers may render your page in quirks mode.
Example Error:
<html>
<head>
<title>Sample</title>
</head>
<body>
<p>Hello World!</p>
</body>
</html>
Fix: Always start your document with the DOCTYPE declaration.
<!DOCTYPE html>
<html>
<head>
<title>Sample</title>
</head>
<body>
<p>Hello World!</p>
</body>
</html>
Common CSS Syntax Errors and Fixes
1. Missing Semicolons
CSS rules are separated by semicolons. Omitting one can break subsequent rules.
Example Error:
body {
color: black
background-color: white;
}
Fix: Add a semicolon at the end of each declaration.
body {
color: black;
background-color: white;
}
2. Misspelled Property Names
Even seasoned developers misspell CSS properties.
Example Error:
body {
bacground-color: white;
}
Fix: Double-check property names.
body {
background-color: white;
}
Pro Tip: Modern browsers’ developer tools can highlight typos.
3. Improper Selectors
Using incorrect or overly specific selectors can lead to ineffective styles.
Example Error:
#main.content > p:first-child
Fix: Use appropriate selectors based on your HTML structure.
#main .content > p:first-child {
font-weight: bold;
}
4. Invalid Units
Using invalid units like pxs
or missing units entirely can cause CSS rules to fail.
Example Error:
p {
font-size: 16;
}
Fix: Specify valid units.
p {
font-size: 16px;
}
Tools to Avoid Syntax Errors
1. Code Editors
Use editors like Visual Studio Code or Sublime Text, which include syntax highlighting and error detection.
2. Validation Tools
- HTML: W3C Validator
- CSS: W3C CSS Validator
3. Browser Developer Tools
Modern browsers like Chrome and Firefox provide built-in tools to inspect and debug HTML and CSS.
Bonus Tips for Better Debugging
- Comment Out Sections: Isolate problematic code by commenting out parts of it.
- Check Browser Console: Look for error messages in the console.
- Test Incrementally: Add styles or elements one at a time to catch errors early.
- Mobile-First Approach: Simplify your CSS by designing for mobile-first and adding complexity progressively.
Wrapping Up
Syntax errors in HTML and CSS can feel like speed bumps on the road to creating stunning websites. But with the right tools, practices, and attention to detail, they’re entirely manageable.
Remember, the key is prevention. Use validation tools, write clean code, and test thoroughly. And if you’re already knee-deep in errors, take a deep breath, grab a coffee, and tackle them one at a time.
Call to Action
Have your own quick fixes or debugging hacks? Share them in the comments! If you found this guide helpful, don’t forget to share it with your fellow developers. Let’s make the web error-free, one tag at a time!