Picture this: You’re a coder, fingers flying over the keyboard, deep in the zone. Then, boom! Your program crashes before it even gets a chance to shine. Or worse, it runs, but something feels… off. Welcome to the world of syntax errors and logic errors—two of the most common headaches in programming. But what exactly sets them apart? Let’s dive in.
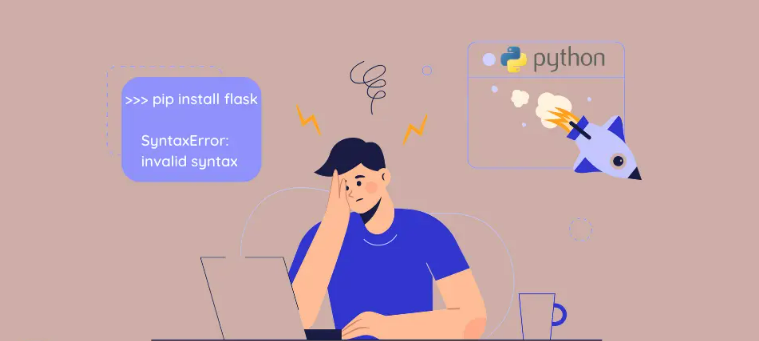
Understanding Syntax Errors
Imagine you’re writing a letter. If you misspell words or forget punctuation, your reader might struggle to understand you. In the world of programming, syntax errors are like these writing mistakes. They occur when you break the rules of the programming language you’re using. Simply put, the computer doesn’t know what you’re trying to say.
Common Causes of Syntax Errors
- Typos: A missing semicolon or a misspelled keyword can derail your entire program.
- Improper structure: Forget to close a parenthesis or bracket? The computer won’t let it slide.
- Wrong order: Mixing up the placement of commands or arguments can leave your code unintelligible.
Real-World Example
Here’s a simple Python snippet:
print("Hello, world!"
See the problem? That missing closing parenthesis is all it takes to trigger a syntax error.
How to Fix Syntax Errors
- Read the error message: Most compilers or interpreters will tell you exactly what’s wrong and where to find it.
- Review your code: Look for typos, missing symbols, or improper formatting.
- Use an IDE: Tools like Visual Studio Code or PyCharm highlight syntax errors in real-time, making them easier to spot.
Understanding Logic Errors
Now let’s move to the stealthier sibling: logic errors. Unlike syntax errors, these don’t prevent your program from running. But the output? It’s wrong, weird, or completely unexpected. Logic errors happen when your code doesn’t do what you intended it to do.
Read more : What Are Syntax Errors? A Beginner’s Guide
Common Causes of Logic Errors
- Faulty algorithms: If the logic behind your solution is flawed, so is your program.
- Incorrect assumptions: Misunderstanding how data flows or functions behave can lead to errors.
- Edge cases: Forgetting to account for special scenarios can break your code.
Real-World Example
Let’s revisit our friend Python:
x = 10
y = 0
result = x / y
print(result)
This won’t throw a syntax error, but you’ll quickly encounter a runtime error (division by zero). Even when logic errors don’t crash the program, they can produce incorrect results—a subtler form of sabotage.
How to Fix Logic Errors
- Debugging tools: Use breakpoints and step-through debugging to watch your code in action.
- Test cases: Run your program with a variety of inputs to ensure it behaves as expected.
- Peer reviews: A second pair of eyes can spot mistakes you’ve overlooked.
Key Differences at a Glance
To make things crystal clear, here’s a quick comparison:
Aspect | Syntax Errors | Logic Errors |
---|---|---|
Definition | Violations of the programming language’s rules. | Flaws in the program’s intended functionality. |
When They Occur | During compilation or interpretation. | During runtime. |
Effect | Prevents the program from running. | Produces incorrect or unexpected results. |
Detection | Easy to spot, as compilers flag them. | Harder to detect without thorough testing. |
Fixing | Requires correcting syntax and structure. | Requires debugging and logical adjustments. |
Why Understanding the Difference Matters
Distinguishing between syntax and logic errors isn’t just academic; it’s essential for becoming an efficient programmer. Here’s why:
- Time management: Knowing the type of error helps you focus on the right solution.
- Improved skills: Recognizing patterns in errors sharpens your coding abilities.
- Fewer headaches: Let’s face it—faster debugging means less frustration.
Tips to Avoid These Errors
While no programmer is immune, you can minimize errors with these strategies:
General Tips
- Write clean code: Use proper indentation and meaningful variable names to make your code easier to read.
- Comment your work: Leave notes explaining complex sections of code for future you (or your teammates).
For Syntax Errors
- Learn the rules: Familiarize yourself with your programming language’s syntax.
- Use a linter: Tools like ESLint or Pylint automatically check your code for issues.
For Logic Errors
- Plan before coding: Map out your algorithms and logic on paper or a whiteboard.
- Unit testing: Break your program into smaller components and test each one independently.
- Continuous learning: Study common algorithmic pitfalls to avoid repeating them.
Wrapping It Up
Syntax errors and logic errors are like the yin and yang of programming mishaps. Syntax errors are loud, obnoxious, and impossible to ignore, while logic errors are the sneaky troublemakers that lurk in the shadows. By understanding their differences and using the right tools and strategies, you can tame both types and become a coding ninja.
So, next time your program misbehaves, take a deep breath, roll up your sleeves, and figure out: Is it a syntax error screaming for attention or a logic error smirking behind the scenes? Either way, you’ve got this.
Call to Action
Found this article helpful? Share it with your fellow coders! And if you have your own war stories about syntax or logic errors, drop them in the comments. Let’s laugh (or cry) together as we conquer the wild world of programming!