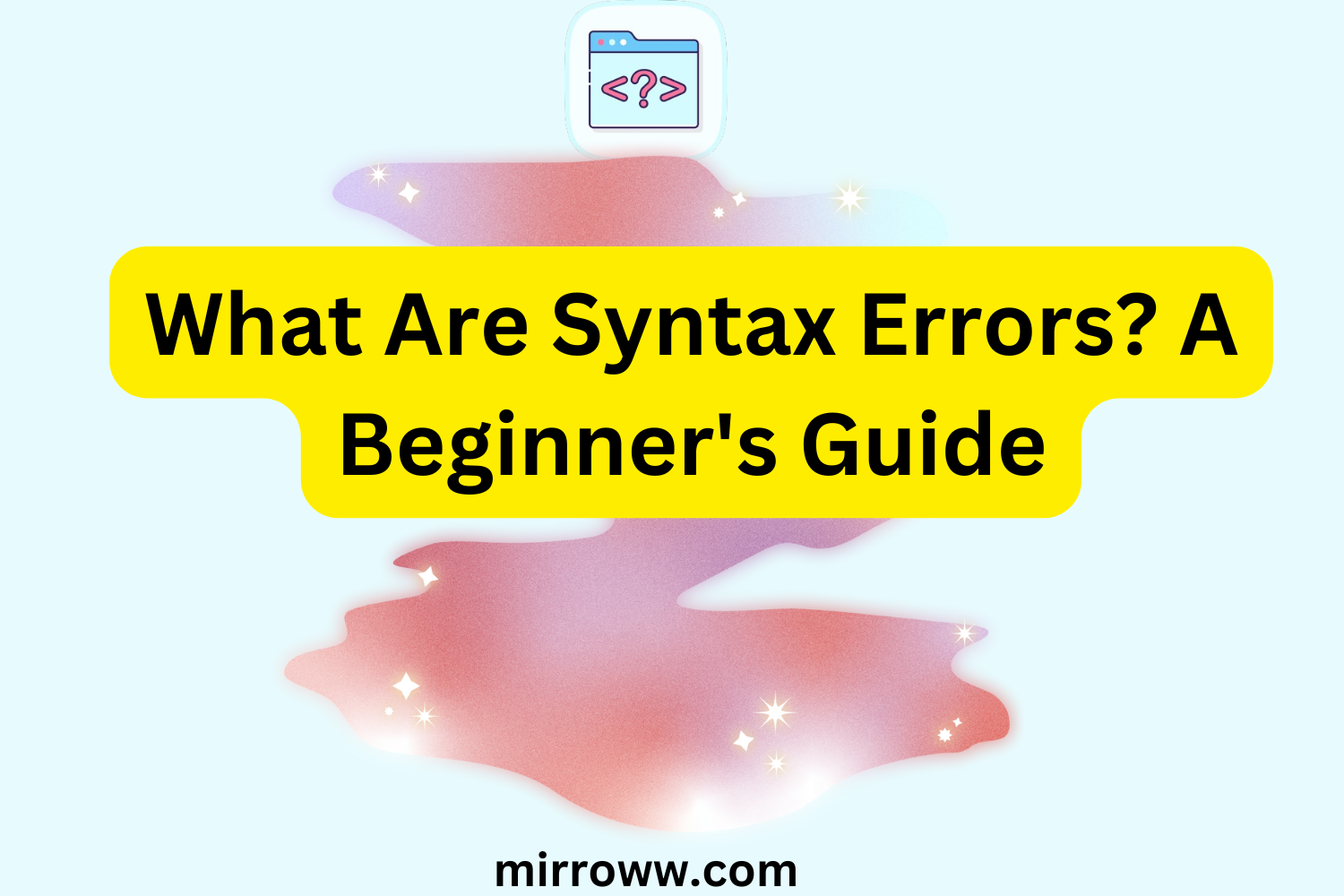
What Are Syntax Errors? A Beginner’s Guide
Imagine you’re a chef, ready to whip up a culinary masterpiece. You gather your ingredients, follow the recipe, but one small detail goes awry—you mistook salt for sugar. Your dish is ruined, leaving you frustrated and wondering where it all went wrong. In programming, syntax errors are like that pesky mix-up in the recipe. They’re the coding equivalent of using salt instead of sugar—a seemingly small mistake that stops your program from running altogether. Let’s dive deeper into the world of syntax errors and uncover why they occur, how to spot them, and most importantly, how to fix them.

What Are Syntax Errors?
In simple terms, a syntax error happens when a programmer breaks the rules of the programming language they’re using. Programming languages are like any other language—they have grammar, punctuation, and structure. Syntax errors occur when there’s a typo, a missing symbol, or anything that doesn’t conform to the strict rules of the language.
Think of it like writing a sentence. If you write, “I going store,” it’s grammatically incorrect. Similarly, in Python, writing print("Hello)
instead of print("Hello")
is a syntax error. The missing closing quotation mark breaks the language’s grammar rules.
Why Do Syntax Errors Happen?
Syntax errors can happen to anyone—from beginners to seasoned developers. Here are some common reasons:
- Typos: A simple misspelling, like writing
pritn
instead ofprint
. - Missing or Extra Characters: Forgetting to close brackets, adding an extra comma, or omitting a colon.
- Incorrect Syntax: Using the wrong format or structure, such as calling a function without parentheses.
- Language-Specific Rules: Every programming language has unique syntax rules, and switching between languages can lead to mistakes.
Real-Life Example: Spotting a Syntax Error
Let’s say you’re writing a Python script to calculate the area of a rectangle:
width = 5
height = 10
area = width * height
print(Area)
Do you see the problem? The variable name Area
in the print
statement doesn’t match the area
variable defined earlier. This small typo will cause Python to throw a syntax error, stopping the program from running.
Common Types of Syntax Errors
1. Mismatched Parentheses, Brackets, or Quotes
- Example:
if (x > 5:
- Fix: Ensure every opening parenthesis or quote has a matching pair.
2. Missing Colons
- Example:
if x > 5 print("Yes")
- Fix: Add a colon:
if x > 5: print("Yes")
3. Misspelled Keywords or Variables
- Example: Writing
funciton
instead offunction
. - Fix: Double-check your spelling.
4. Improper Indentation (in languages like Python)
- Example:
def my_function(): print("Hello")
- Fix: Ensure proper indentation:
def my_function(): print("Hello")
5. Extra Commas or Periods
- Example:
[1, 2, 3,]
- Fix: Remove the extra comma:
[1, 2, 3]
How to Spot and Fix Syntax Errors
1. Use an IDE or Code Editor
Modern Integrated Development Environments (IDEs) and code editors, like VS Code or PyCharm, highlight syntax errors in real time. They often provide hints or suggestions to fix the issue.
2. Read Error Messages Carefully
When you run your code and encounter a syntax error, read the error message closely. It usually indicates where the error occurred and what went wrong.
3. Check Line by Line
If the error message isn’t clear, review your code line by line. Look for typos, mismatched symbols, or formatting issues.
4. Use Debugging Tools
Debugging tools can help you step through your code and identify the exact point where it breaks.
5. Take a Break
Sometimes, the best way to find a syntax error is to step away and return with fresh eyes. You’d be surprised how quickly you can spot the issue after a short break.
The Frustration (and Humor) of Syntax Errors
Anyone who’s spent hours hunting down a missing semicolon or an extra comma knows the struggle is real. Syntax errors can be infuriating, but they’re also a rite of passage for every programmer. They teach you to pay attention to detail and, eventually, to laugh at your mistakes. After all, who hasn’t accidentally created infinite loops or forgotten a single curly brace?
The Silver Lining
Here’s the good news: syntax errors are easy to fix once you spot them. They don’t require complex debugging or deep knowledge of algorithms. They’re simply reminders that computers are sticklers for rules. Fix the error, and you’re back in action.
Preventing Syntax Errors
To minimize syntax errors:
- Practice Regularly: Familiarity with a programming language reduces mistakes.
- Use Linting Tools: These tools analyze your code for potential issues and suggest improvements.
- Follow a Style Guide: Consistent coding practices make errors easier to spot.
- Learn from Mistakes: Every syntax error is a lesson. Take note of common pitfalls and avoid repeating them.
Final Thoughts
Syntax errors may seem like the villain of your programming journey, but they’re really just tough love from your computer. They push you to write cleaner, more precise code. So the next time you encounter a syntax error, take a deep breath, channel your inner detective, and remember: every great programmer started with syntax mistakes.
Got a syntax error story to share? Let us know in the comments below. And if you’re new to coding, keep at it. The more you practice, the fewer errors you’ll make. Happy coding!
Call to Action
Found this guide helpful? Share it with your fellow coding enthusiasts! For more beginner-friendly programming tips and tutorials, subscribe to our newsletter. Let’s tackle the world of code together!